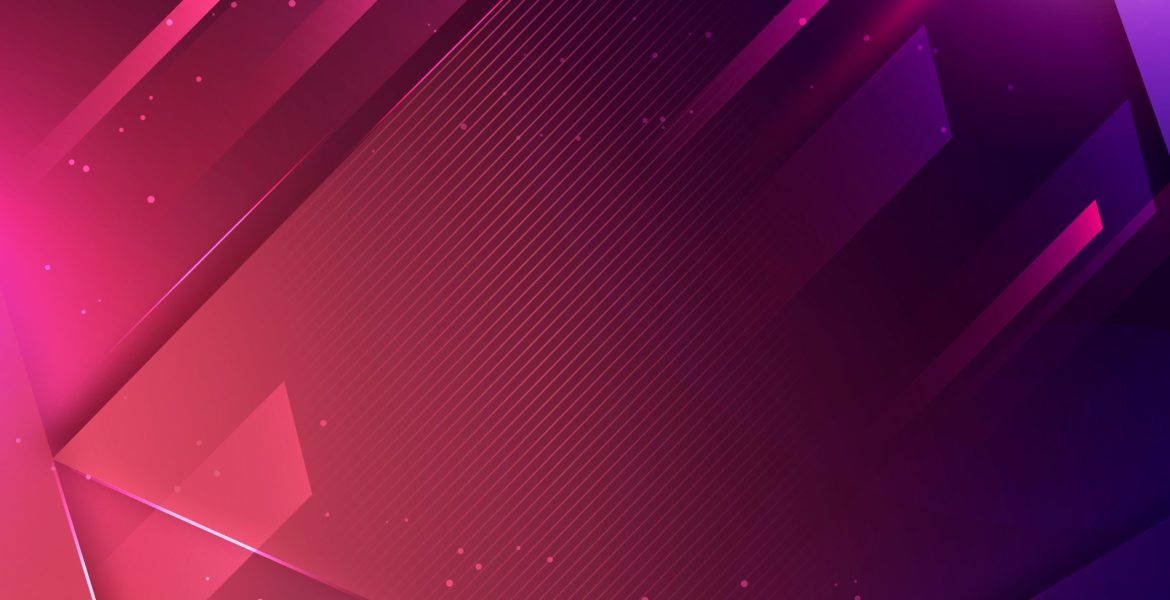
NestJS is a popular open-source framework that is used for building scalable and efficient server-side web applications. It is built on top of Node.js and uses TypeScript, which makes it an excellent choice for developing large-scale enterprise-level applications. In this blog, we will discuss how to build web applications with NestJS.
Getting Started with NestJS
Before we begin, let’s first install NestJS. You can use the following command to install NestJS globally:
npm install -g @nestjs/cli
Once you have installed NestJS, you can create a new project using the following command:
nest new myproject
This will create a new NestJS project with a basic directory structure.
Creating Controllers
Controllers are responsible for handling incoming HTTP requests and returning responses to the client. To create a new controller, use the following command:
nest generate controller mycontroller
This will create a new controller file in the src
directory. You can define your controller methods in this file, like so:
import { Controller, Get } from '@nestjs/common';
@Controller('my')
export class MyController {
@Get()
myMethod(): string {
return 'Hello World!';
}
}
In this example, we are creating a new controller called MyController
that responds to HTTP GET requests at the /my
endpoint. The myMethod
method returns the string Hello World!
when called.
Creating Services
Services are responsible for handling business logic and performing data operations. To create a new service, use the following command:
nest generate service myservice
This will create a new service file in the src
directory. You can define your service methods in this file, like so:
import { Injectable } from '@nestjs/common';
@Injectable()
export class MyService {
myServiceMethod(): string {
return 'This is my service method!';
}
}
In this example, we are creating a new service called MyService
that has a single method called myServiceMethod
. This method returns the string This is my service method!
when called.
Injecting Services into Controllers
To use a service inside a controller, we need to inject it into the controller using the @Injectable
decorator. Here’s an example:
import { Controller, Get } from '@nestjs/common';
import { MyService } from './my.service';
@Controller('my')
export class MyController {
constructor(private readonly myService: MyService) {}
@Get()
myMethod(): string {
return this.myService.myServiceMethod();
}
}
In this example, we are injecting the MyService
service into the MyController
controller using the private readonly
syntax. The myMethod
the method then calls the myServiceMethod
method of the MyService
service and returns its result.
Conclusion
In this blog post, we discussed how to build web applications with NestJS. We covered the basics of creating controllers and services, injecting services into controllers, and responding to HTTP requests. With these concepts in mind, you should now be able to start building your own web applications using NestJS.
Recent Comments