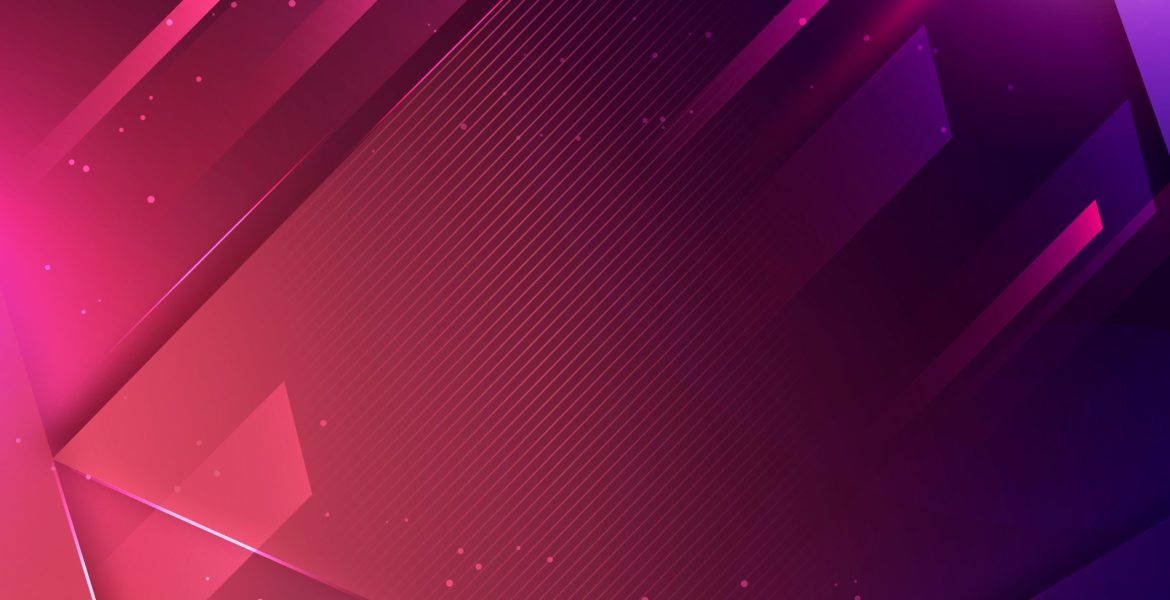
Modern web development is all about creating interactive, efficient, and scalable applications. Two technologies that stand out in this respect are React.js and Node.js. React is a powerful JavaScript library for building dynamic user interfaces, while Node.js provides a runtime environment to execute JavaScript on the server side.
When used together, React.js and Node.js can create a robust full-stack JavaScript environment that’s perfect for modern web application development. In this blog, we’ll discuss how to build web applications using these two powerful technologies.
React.js: A Brief Overview
React.js, often simply referred to as React, was developed by Facebook and is used to build user interfaces, particularly for single-page applications. It allows developers to create reusable UI components, leading to more efficient, readable code that’s easier to maintain.
React uses a virtual DOM, which significantly improves app performance. The virtual DOM updates parts of the web page without having to reload the entire page. This results in a smoother and faster user experience.
Node.js: A Brief Overview
Node.js is a JavaScript runtime built on Chrome’s V8 JavaScript engine. It allows developers to execute JavaScript code on the server side. With Node.js, you can build scalable network applications that can handle a high number of simultaneous connections with high throughput.
Node.js follows an event-driven, non-blocking I/O model, which makes it lightweight and efficient. It’s perfect for data-intensive real-time applications that run across distributed devices.
Setting Up the Development Environment
To start building applications with React.js and Node.js, you need to have Node.js and npm (Node Package Manager) installed on your machine. npm is automatically installed when you install Node.js. It is the world’s largest software registry, and it’s what you’ll use to install React.js and other JavaScript packages.
Once Node.js and npm are installed, you can install the create-react-app
package, a tool that sets up a new React project with a good default configuration. You can install it globally with the following command:
npm install -g create-react-app
Creating a React.js Frontend
Once you’ve installed create-react-app
, you can create a new React app by running:
npx create-react-app my-app
This creates a new directory called my-app
with all the boilerplate code you need to get started. You can then navigate into your my-app
directory and start the development server:
cd my-app
npm start
The npm start
command starts a development server that serves your React application. It also automatically opens the application in your default web browser.
Creating a Node.js Backend
On the backend, you can use Node.js with Express.js, a minimal and flexible Node.js web application framework. First, let’s create a new directory for the backend application and initialize it with npm:
mkdir my-api
cd my-api
npm init -y
This will create a package.json
file in your project root. Next, install Express:
npm install express
To create a simple server, you can create an app.js
file in your root directory and add the following code:
const express = require('express');
const app = express();
const port = 3001;
app.get('/api', (req, res) => {
res.json({ message: 'Hello from server!' });
});
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
This code creates a new Express application and starts it listening on port 3001. It also defines a single route (/api
) that returns a JSON response with a welcome message.
You can start your Node.js server by running:
node app.js
Connecting Frontend and Backend
With the frontend and backend servers running, the next step is to make them communicate with each other. One common way is to have your React app make HTTP requests to your Node.js server.
To do this, you might use a library like axios
or the built-in fetch
API. Let’s use axios
as an example:
First, install it in your React application:
cd ../my-app
npm install axios
Then, you can use axios
to send a GET request to your Node.js server:
import axios from 'axios';
axios.get('/api')
.then(response => {
console.log(response.data.message);
})
.catch(error => {
console.error(`Error: ${error}`);
});
Here, we’re sending a GET request to the /api
route we defined in our Node.js server and logged the response message.
One thing to note is that your React app and Node.js server are running on different ports, which means they are on different origins. Therefore, trying to make a request from the React app to the Node.js server would be a cross-origin request, which is blocked by the browser’s same-origin policy.
To get around this, you can use a feature of create-react-app
called proxying API requests. In your package.json
file, you can add a proxy
a field like so:
{
"name": "my-app",
"version": "0.1.0",
"proxy": "http://localhost:3001",
...
}
Now, when you make a request to /api
from your React app, it’ll automatically be forwarded to http://localhost:3001/api
.
Conclusion
And there you have it – a basic full-stack web application using React.js for the front end and Node.js for the backend! React and Node.js are powerful tools for building modern, scalable, and efficient web applications. With these tools in your toolkit, you’ll be well-equipped to tackle a wide variety of web development projects.
Remember, what we’ve covered here is just the tip of the iceberg. Both React and Node.js have large ecosystems with plenty of libraries and frameworks that can help you build more complex, feature-rich applications. Happy coding!
Recent Comments