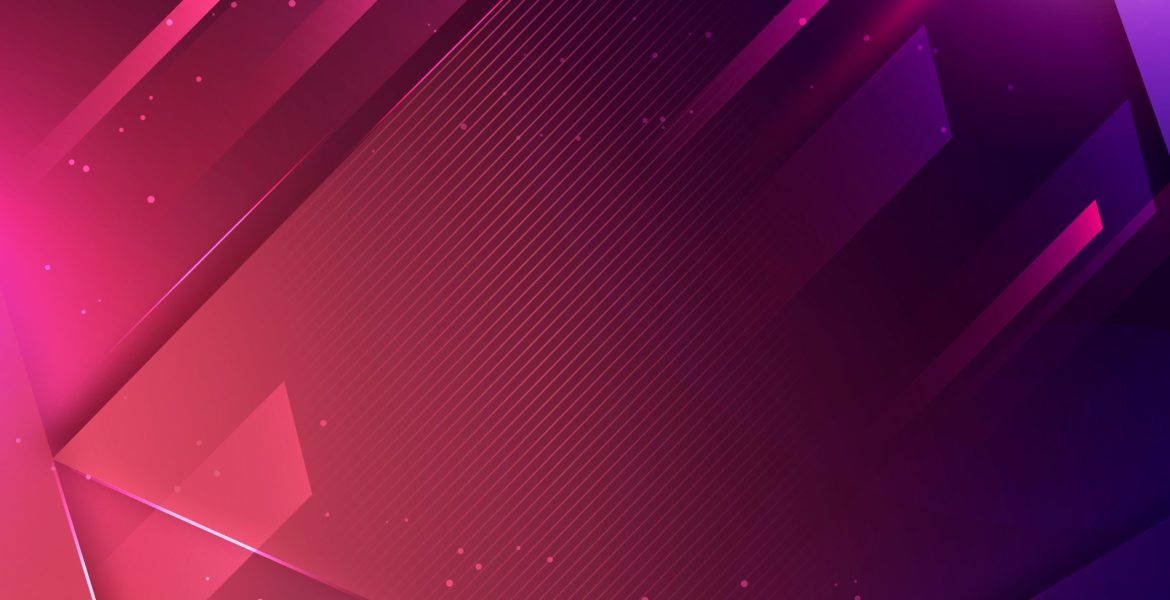
AngularJS is a popular JavaScript framework for building web applications. It is an open-source framework maintained by Google and provides a set of tools and features that make it easy to build dynamic, responsive web applications. In this blog post, we will explore the steps involved in building web applications with AngularJS.
1. Setup AngularJS
To get started, you need to download and install AngularJS. You can download the latest version of AngularJS from the official website. Once you have downloaded AngularJS, you can set up your development environment. You will need a text editor or an Integrated Development Environment (IDE) such as Visual Studio Code or WebStorm.
2. Define the App Module
The first step in building a web application with AngularJS is to define the app module. The app module is the root module of your application and defines the components, directives, and services that your application will use. You can define the app module in a JavaScript file by calling the angular.module function.
var app = angular.module('myApp', []);
In the above code, we define a module named ‘myApp’ with an empty array of dependencies. You can add dependencies to this array as needed.
3. Define Controllers
Controllers are responsible for handling user interactions and updating the view. You can define a controller in a JavaScript file by calling the controller function.
app.controller('myCtrl', function($scope) {
$scope.name = "John Doe";
});
In the above code, we define a controller named ‘myCtrl’ that sets the value of the name variable to “John Doe”. You can add more properties and functions to the $scope object as needed.
4. Define Directives
Directives are used to extend HTML with custom elements and attributes. You can define a directive in a JavaScript file by calling the directive function.
app.directive('myDirective', function() {
return {
template : "<h1>Hello World!</h1>"
};
});
In the above code, we define a directive named ‘myDirective’ that replaces the element with the template “<h1>Hello World!</h1>”.
5. Define Services
Services are used to share data and functionality across components. You can define a service in a JavaScript file by calling the service function.
app.service('myService', function() {
this.getData = function() {
return "Some data";
};
});
In the above code, we define a service named ‘myService’ that returns the string “Some data” when the getData function is called.
6. Use AngularJS Directives and Expressions
Once you have defined the app module, controllers, directives, and services, you can start using them in your HTML code. You can use AngularJS directives and expressions to bind data, events, and properties to your HTML elements.
<div ng-app="myApp" ng-controller="myCtrl">
<h1>{{name}}</h1>
<my-directive></my-directive>
</div>
In the above code, we use the ng-app directive to define the app module, the ng-controller directive to define the controller, and the {{name}} expression to bind the value of the name variable to the H1 element. We also use the <my-directive> element to render the directive defined earlier.
7. Test and Debug Your Application
Finally, you can test and debug your application using the developer tools in your browser. You can use the console to log errors, inspect the scope, and monitor network requests. You can also use the built-in testing framework in AngularJS to write and run tests for your application.
In conclusion
AngularJS is a powerful framework that provides a comprehensive set of tools and features for building web applications. By following the steps outlined above, you can build dynamic, responsive web applications with AngularJS. However, there are some best practices that you should keep in mind when using AngularJS, including:
- Use the latest version of AngularJS.
- Follow the AngularJS style guide.
- Use dependency injection to manage dependencies.
- Use services to share data and functionality.
- Use directives to extend HTML.
- Use controllers to handle user interactions.
- Use filters to transform data.
- Use templates to define views.
- Use routing to navigate between views.
- Use testing to ensure the quality of your application.
By following these best practices, you can ensure that your AngularJS application is well-organized, maintainable, and scalable. You can also leverage the vast ecosystem of AngularJS libraries and tools to enhance your application and improve your development workflow. With its robust set of features and tools, AngularJS is an excellent choice for building web applications that are both powerful and flexible.
Recent Comments